EZ Message Manager is the beginning of a web application to schedule sending emails & slack messages to employees based on dynamic queries. I am sharing the features, lessons learned, and the source code.
EZMessageManager has three parts, the frontend, backend, and cron.
History
I needed to schedule recurring messages to new employees about onboarding. For the past couple of months, I have been teaching myself React & Nodejs in my free time. I fell in love with a headless CMS concept and wanted to build a react frontend application on top of a Nodejs using a Strapi backend with Restful API and GraphQL.
I worked with a freelance developer to help expedite the development process, but since we were both novice nodejs/react developers, the code still needs more love. Given this was a “learning” project for me, I thought it would be best to share it with the world as open-source and allow other developers to learn or contribute their genius.
Technology Stack
- Nodejs/React
- Material-UI
- Strapi - A headless CMS using a PostgreSQL database, with queries handled via Strapi REST API endpoints or Strapi GraphQL with React Apollo.
- Devias Front-end Admin Template
- Unlayer - A free email editor for building responsive email templates.
- Mailtrap - For SMTP testing.
- PM2 — For mail and Slack message scheduling.

Features
- Manage Employees: Assign tags and add metadata fields based on unique employee information (e.g., demographic, organization, manager, expertise).
- Email Templates: Generate email templates using Unlayer, with the ability to include employee profile and metadata fields.
- Contact List: Build dynamic distribution lists of employees based on GraphQL queries.
- Email Schedules: Schedule emails to be sent to a contact list using an email template.
- Slack Schedule: Schedule Slack messages to a contact list using an email template.
- JWT Authentication: Manage users and accounts using Strapi’s built-in JWT authentication.
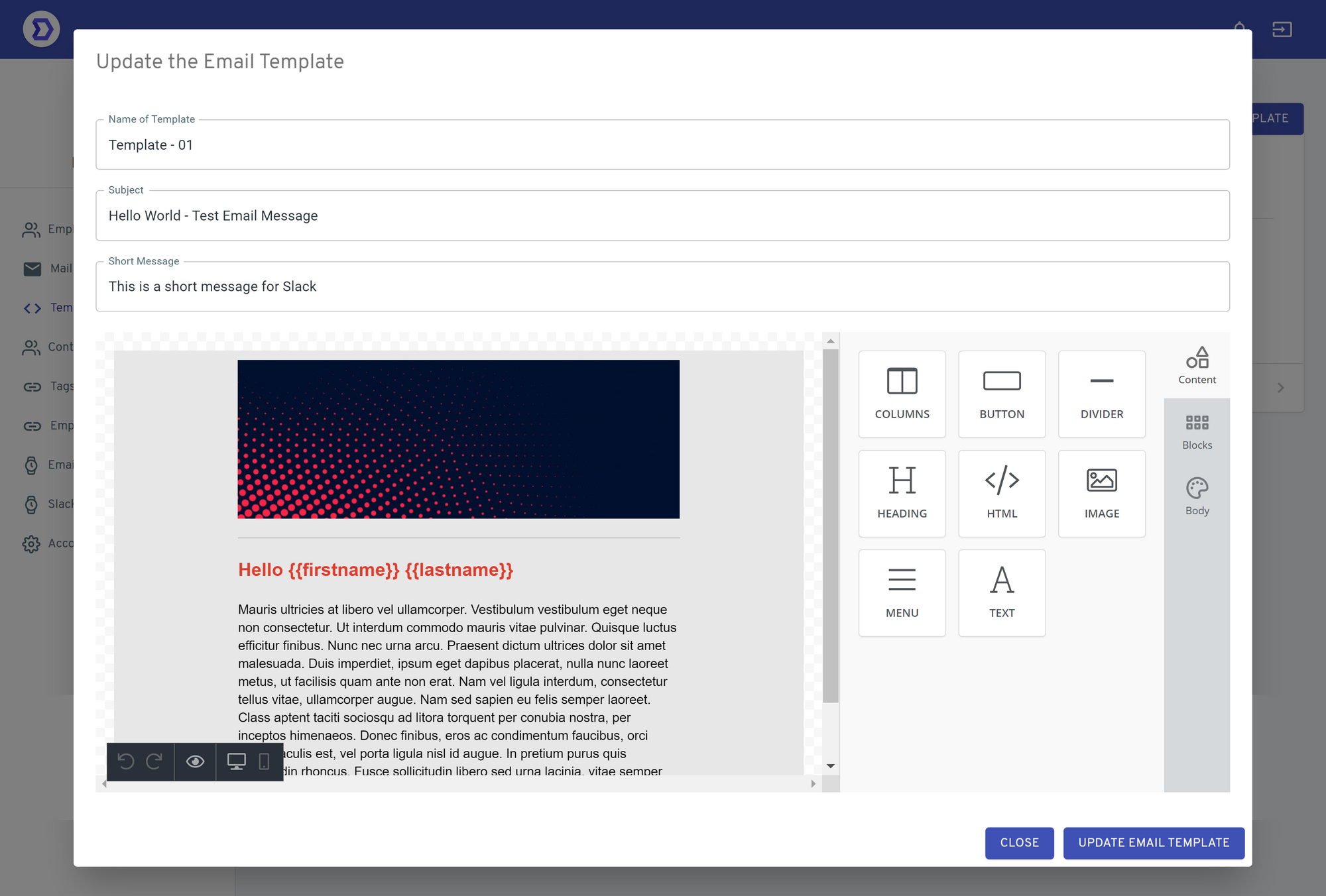
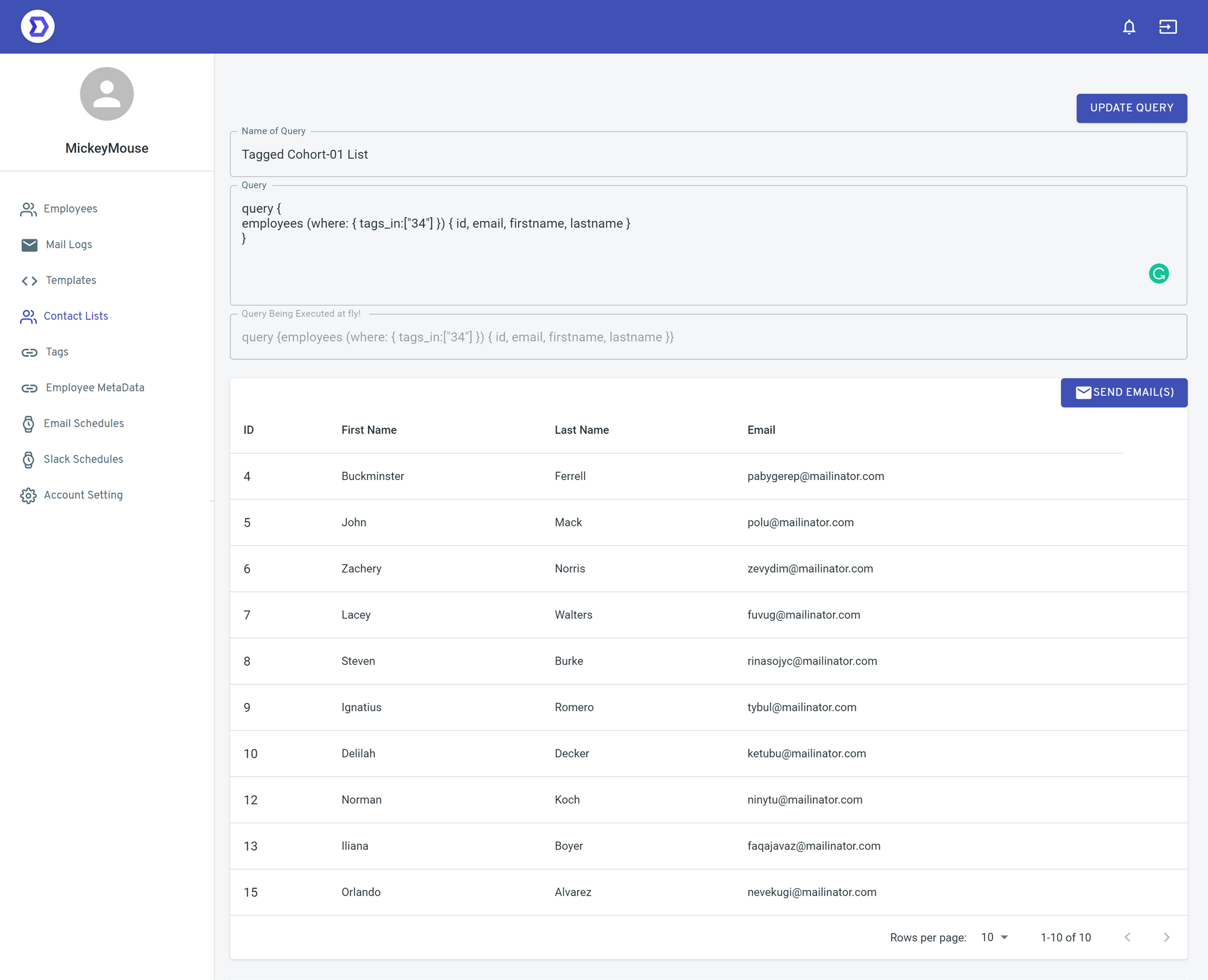
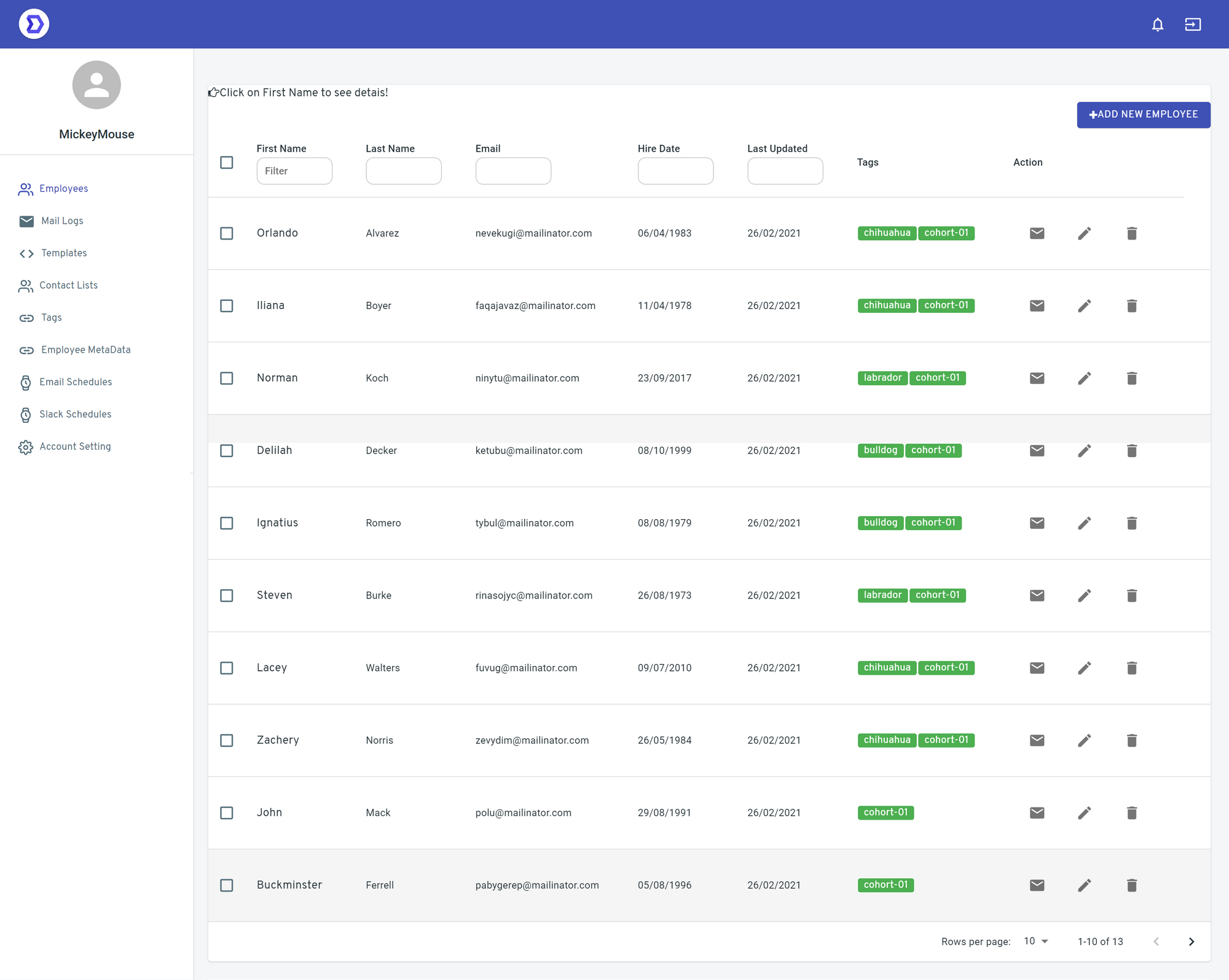
How it Works
Backend - https://github.com/jazmy/ezmessagemanager-backend
The backend (Strapi running on Node.js) can be easily deployed on Heroku and connected directly to GitHub for automatic builds and deploys. It is recommended to fork the GitHub repository for your own version to connect to a free Heroku account. Note: Using the free version of Heroku, which falls asleep after 30 minutes, is not ideal for this application as scheduled emails may fail, but it works fine for testing.
Frontend - https://github.com/jazmy/ezmessagemanager-frontend
The frontend is deployed on Netlify and connected directly to the GitHub repository for automatic builds and deploys, using their free tier.
Cron - https://github.com/jazmy/ezmessagemanager-cron
The cron uses PM2, a production process manager for Node.js. A procfile, ecosystem.config.js, and updates to the package.json start script enable seamless deployment on Heroku.
SMTP - Configured to use Mailtrap for testing
Contact Lists
Typically, contact lists require manual upload of employee data. However, to automatically send emails to everyone in a specific organization or hired within a certain timeframe, I needed a dynamic solution. The project allows creating queries that run each time a scheduled email is triggered, ensuring the most up-to-date list of employees based on defined criteria.
The contact lists are stored as GraphQL queries in the database and are executed each time an email is scheduled to send. If I were to rewrite this, I would utilize Strapi’s built-in Strapi restful API filters.
Example Query
To create a contact list, a GraphQL query is required.
Example: This query looks for employees hired after 2010-01-08 but before 2021-01-14 and assigned the tag with an id: 2.
query {
employees (where: { hiredate_gt: "2010-01-08", hiredate_lt: "2021-01-14", tags_in:["2"] }) {
id, email, firstname, lastname
}
}
Challenge: GraphQL does not support “Now” variables for the current date/time. My workaround involved parsing the query with regular expressions to replace the word “today” with the current date, and adjusting by a numeric value in “brackets” to add or subtract days.
Hired in the last 90 days:
query {
employees (where: { hiredate_gte: "today",tags_in:["1"] }) { id, email, firstname, lastname }
}<-90>